12/11/2009 - Update for Managed Code.
1.0 Introduction
For some time now I have been pretty unhappy with InfoPath deployment. I really did not know of a way to deploy InfoPath forms other than publishing them using the InfoPath Designer. I was even more frustrated with having to go to SharePoint Central Administration to deploy a form when I had to give the form Full Trust. Sometimes I had to give Full Trust to InfoPath sometimes for making data connections. As well, I would purposely avoid adding managed code at all cost to my InfoPath forms (still if I have to put in managed code into my InfoPath form, I should use ASP.net - InfoPath and ASP.net considerations).
Well someone made a comment to my blog which showed me the light – YES you can deploy an InfoPath form as a Feature. Awesome! Totally Awesome! Some may say this is old news, but I really just wanted to share the knowledge on this one.
I am excited about deploying InfoPath as a Feature for the following reasons:
- The InfoPath form will be deployed as a content type. I have many scenarios where I have to publish an InfoPath form to many form libraries. For instance I have business process where the InfoPath form will be initiated in one Form Library and archived in a different one. Now I do not have to go to each form library and redeploy. Since it is a content type that will be added to a Form Library, when I redeploy the form with updates, all of the form libraries are updated.
- The form gets installed into Central Admin requiring me less clicking and less steps.
- I can now automate the deployment of my InfoPath forms and package them up in .wsp!!! That is really where I wanted to get to.
2.0 Feature
Getting to the fun stuff first, the following is a feature that will deploy your InfoPath form for you.
2.1 Feature file
<?xml version="1.0" encoding="utf-8" ?>
<Feature xmlns="http://schemas.microsoft.com/sharepoint/"
Id="DE7C8197-4FF3-41f5-B13A-6ACB94FA0C77"
Title="New User Request Form"
Description="This feature deploys the browser enabled New User Request InfoPath Form."
Version="12.0.0.0"
Scope="Site"
DefaultResourceFile="ipfscore"
ReceiverClass="Microsoft.Office.InfoPath.Server.Administration.XsnFeatureReceiver"
ReceiverAssembly="Microsoft.Office.InfoPath.Server, Version=12.0.0.0, Culture=neutral, PublicKeyToken=71e9bce111e9429c" >
<ActivationDependencies>
<ActivationDependency FeatureId="C88C4FF1-DBF5-4649-AD9F-C6C426EBCBF5"/>
</ActivationDependencies>
<ElementManifests>
<ElementManifest Location="element.xml"/>
<ElementFile Location="NewUserRequestForm.xsn"/>
</ElementManifests>
</Feature>
2.2 Element File
<?xml version="1.0" encoding="utf-8" ?>
<Elements xmlns="http://schemas.microsoft.com/sharepoint/">
<Module Name="XSN" Url="FormServerTemplates" RootWebOnly="TRUE">
<File Url="NewUserRequestForm.xsn"
Name="NewUserRequestForm.xsn"
Type="GhostableInLibrary"/>
</Module>
</Elements>
Notes:
- The key to this whole thing is the receiver class XsnFeatureReceiver.
- In the Feature File the Enterprise Feature is turned on by creating an activation dependency on C88C4FF1-DBF5-4649-AD9F-C6C426EBCBF5.
- The name of the InfoPath form is an ElementFile in the Feature.xml and is a Module in the Element.xml.
3.0 Create Project
The following is what I had to do create a project for the InfoPath form.
3.1 Visual Studio
- I created a Visual Studio C# project. Yes, I still believe in being able to do some of this stuff from scratch.
- In the project I added a TEMPLATE, FEATURE and a feature Folder called K2Distillery.IP.NewUserForm. In the Feature folder I put the element.xml and feature.xml. The InfoPath form will be published to Feature Form later. I explain why I do this a little farther down.
- In the project, I deleted the default class that was created and I added the InfoPath form into the root of the project.
- I created a file called WSP.ddf in the root of the project. This will be used to create a solution package to deploy and InfoPath Feature.
- I then finally added a manifest.xml file to the project.
- In the properties of the project, in the Post Build Events, I added the following which will create my deployment package when the project is built:
cd $(ProjectDir)
MakeCAB /D outputDir=$(OutDir) /f "WSP.ddf"
The following is what the project solution looked like when I was completed.
3.2 manifest.xml File
The manifest.xml file is used by the WSP solution package to deploy the Feature.
<Solution xmlns="http://schemas.microsoft.com/sharepoint/"
SolutionId="F9D4D78A-06AA-4625-9676-0A27E6299E05">
<FeatureManifests>
<FeatureManifest Location="K2Distillery.IP.NewUserForm\feature.xml"/>
</FeatureManifests>
</Solution>
3.3 WSP.ddf File
This file is used create the WSP file that will be deployed into SharePoint. The great thing about doing this is if there are other Features I need to deploy along with this InfoPath Form Feature, I can package them up in this single solution and all of the files will be deployed across the entire farm. For instance, if I really want to get slick, I could create a different feature to create a Form Library and then write a Feature receiver to get the content type for the InfoPath form and associate it to the Form Library on the fly.
I highly recommend that you get into this habit. It is not that hard once you learn it.
.OPTION Explicit
.Set CabinetNameTemplate="K2Distillery.IP.NewUserForm.wsp"
.Set DiskDirectory1="C:\K2Distillery\IP\K2Distillery.IP\K2Distillery.IP.NewUserForm"
manifest.xml
.Set DestinationDir="K2Distillery.IP"
.Set DestinationDir="K2Distillery.IP.NewUserForm"
TEMPLATE\FEATURES\K2Distillery.IP.NewUserForm\element.xml
TEMPLATE\FEATURES\K2Distillery.IP.NewUserForm\feature.xml
TEMPLATE\FEATURES\K2Distillery.IP.NewUserForm\NewUserRequestForm.xsn
.Delete outputDir
4.0 Deployment Steps
The next step is to deploy the solution. I have two steps.
4.1 InfoPath Deployment
You may have noticed in my project I have the InfoPath form in the root of the project and in the Feature folder. You will see what I did this in a moment.
- Open the InfoPath form in the root of the project in InfoPath Designer.
- Select File >> Publish:
- To Network Location
- In the next step, navigate to the \\TEMPLATE\FEATURES\K2Distillery.IP.NewUserForm folder.
- In this step of the publishing process, clear this text box and press the Next button. This is the reason why I have two different copies of the InfoPath form in the project. The first one is the Form I make modifications to and the second one is the form that will actually be deployed to MOSS.
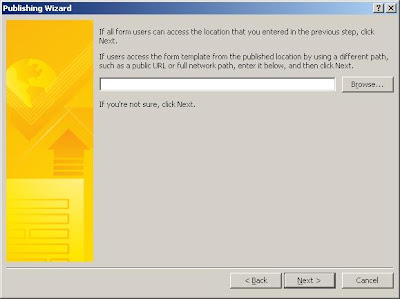
- Then Finish the wizard and close InfoPath.
4.2 Solution Deployment
- Since the manifest.xml and WSP.ddf files have been added to the project and I have added Post Build commands to generate the WSP file, the only thing I need to do is build Project. Easy.
- Once the project has been built a file called K2Distillery.IP.NewUserForm.wsp should have be created. The location of it is in the WSP.ddf file.
- Then all I need to do is deploy this using stsadm.exe:
stsadm.exe -o addsolution -filename C:\K2Distillery\IP\K2Distillery.IP\K2Distillery.IP.NewUserForm\K2Distillery.IP.NewUserForm.wsp
stsadm.exe -o deploysolution -name K2Distillery.IP.NewUserForm.wsp -immediate -force
stsadm.exe -o execadmsvcjobs
- Then finally I need to activate the Feature on the site:
stsadm.exe -o activatefeature -filename K2Distillery.IP.NewUserForm\feature.xml -url %SITEURL% -force
All of these steps could have been done through Central Administration and in Site Settings however, going down this path you will be able to create one touch deployment command files that can deploy your entire solution into a production environment.
4.3 Verifying Deployment
There are several places for you to verify that everything went well:
- Go to Central Administration and you will see that you form has been added there.
- Go to the site/FormServerTemplates/ and you will see the form there.
- Go to Site Settings and you should find a new Content Type called NewUserRequestForm has been created. The InfoPath form will be set up as the document template for the Content Type.
4.4 Adding the Form to a Form Library
It is pretty common knowledge on how to add Content Type for a List, Document Library or Form Library. Well the steps have not changed. Create a Form Library, go to the form settings, go to advanced settings, allow management of content types and then add the content type for the form to the Form Library.
4.5 Promoted Fields
If you are wondering where are the Promoted Fields? Well they are there, however they are not added as a column to the Form Library when you associate the Content Type. At first I did not know where they were because I was accustomed to having promoted fields added as columns of the Form Library automatically because when I publish a form using InfoPath Designer. To add them, all you need to do is:
- Go to the Form Library settings.
- Scroll down to the Views section at the bottom.
- Click on a view.
- Check the promoted InfoPath form field which will be listed as a column. That is it.
Yes, I could probably create another Feature to do this for me too, but at this point, I need to move on J
4.6 Managed Code
Read the following posting I wrote after writing this blog.
5.0 Conclusions
Some may say you had to do just as much effort, if not more, to deploy the solution in this manner versus deploy the form using InfoPath Designer or through Central Administration. It really comes down to following a true publishing model with SharePoint. Many say if you cannot deploy something as a Feature it should not be deployed at all. I agree with this sentiment in many respects (and why I avoid using SharePoint Designer). I do agree there is a point where some manual configuration is required to deploy between SharePoint environments.
References
Deploy InfoPath Form as a Feature – Yes, I had a little help here J